이번 lecture에서 배우게 될 내용은 아래와 같다.
1) Add mongoose into app.js
2) connect mongoose and mongodb
3) setup campgroundSchema
4) compile schema into model
5) /campground route로 연결될 경우 db에서 조회
6) get data from POST /campgrounds and save into mongo
해당 소스는 이전에 작성한 YelpCamp/v1을 v2로 복사하여 사용한다.
app.js
const express = require("express");
const app = express();
const bodyParser = require("body-parser");
const mongoose = require("mongoose");
// Connection between mongoose and mongodd
// 기존의 UrlParser가 사라질 예정이므로 다음과 같은 에러 발생 시 line 10의 옵션을 사용. DeprecationWarning: current URL string parser is deprecated, and will be removed in a future version. To use the new parser, pass option { useNewUrlParser: true } to MongoClient.connect.(node:10712) DeprecationWarning: current Server Discovery and Monitoring engine is deprecated, and will be removed in a future version. To use the new Server Discover and Monitoring engine, pass option { useUnifiedTopology: true } to the MongoClient constructor.
mongoose.connect("mongodb://localhost/yelp_camp" , {userNewUrlParser: true, useUnifieldTopology: true});
// express가 bodyParser를 사용하도록 package 호출
app.use(bodyParser.urlencoded({extended: true}));
// ejs package 호출 (사용 시 ejs 확장자 입력하지 않아도 됨)
app.set("view engine", "ejs");
// MongoDB에서 사용할 Schema setup
const campgroundSchema = new mongoose.Schema({
name: String,
image: String
});
// line 18에서 생성한 campgroundSchema를 컴파일하여 mongoose에서 모델링 후 Campground라는 변수에 저장
const Campground = mongoose.model("Campground", campgroundSchema);
app.get("/campgrounds", (req, res) => {
// 해당 route에서 보여줄 데이터를 MongoDB로부터 가져온다.
Campground.find({}, (err, allCampgrounds) => { //Campground라는 collection에서 모든 데이터를 가져온다
if(err) {
console.log(err)
} else {
res.render("campgrounds", {campgrounds: allCampgrounds});
}
})
});
// 클라이언트에서 입력한 데이터를 DB에 저장하는 부분
app.post("/campgrounds", (req, res) => {
// 클라이언트에서 입력한 name과 image를 변수에 저장
const name = req.body.name;
const image = req.body.image;
// 위의 name과 image를 newCampgroud라는 object에 저장
const newCampground = {name: name, image: image};
// Create a new campground and save into DB
Campground.save({newCapground, (err, newlyCreated) => {
if(err) {
console.log(err);
} else {
// 저장 후 결과를 보여줄 수 있도록 redirect to campground page
res.redirect("campgrounds");
}
})
});
app.get("/campgrounds/new", (req, res) => {
res.render("new.ejs");
});
app.get("/", (req, res) => {
res.render("landing");
});
app.listen(process.env.PORT || 3000, => {
console.log("The YelpCam v2 server has been started");
});
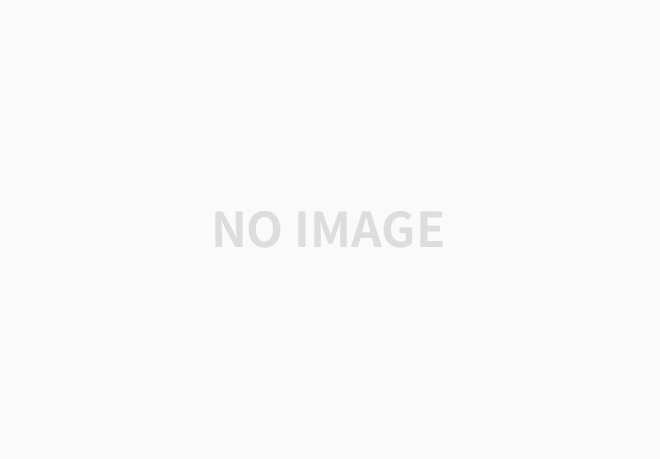